MINI, an extremely simple barebone PHP application
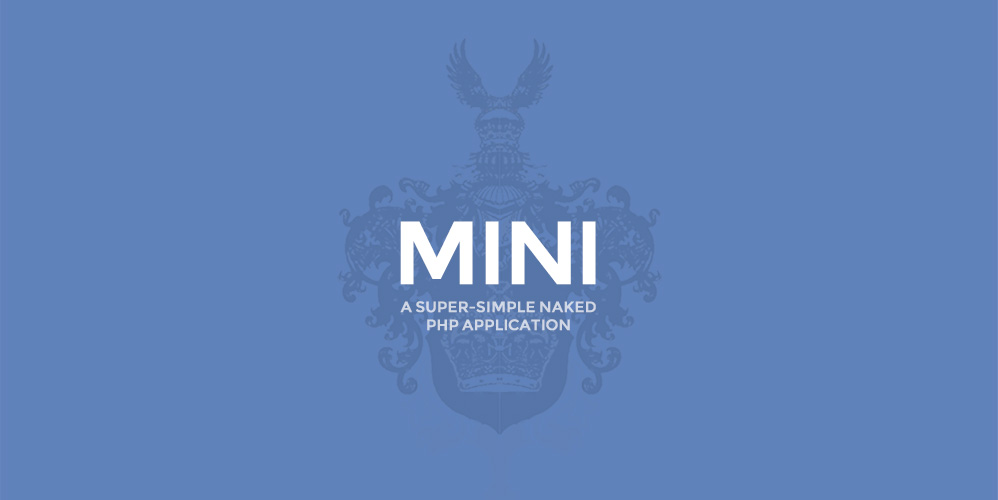
For my daily work I often needed to setup super-simple PHP applications, just some more or less static pages plus some dynamic pages with simple database calls, one or two simple forms and maybe a little bit of AJAX. You know, the typical agency stuff. This usually led to the question: Use a real framework or just mash some .php files together ? Building a simple structure from scratch was painfully stressful every time (and usually also very messy and unsecure), using a real framework was also annoying as setting them up and reading into the documentation to do the most simple tasks took lot of time. And let’s be honest, we probably all have gone through feature-hell in frameworks, I still remember trying to get decorators in Zend Framework 1 to work, for WEEKS, when a simple echo $myvariable would have done the job in seconds perfectly. So I’ve build a very very simple application skeleton, some might even call it a framework, that tries to do common PHP application tasks in the most simple way possible, in pure native PHP, without any bullshit around: MINI.
The features of MINI
- an extremely simple, easy to understand barebone PHP application, for the most simple use-cases
- has a very simple but clean structure
- makes “beautiful” clean URLs (like example.com/car/show/17, not example.com/index.php?type=car&action=show&id=17)
- demo CRUD actions: Create, Read, Update and Delete database entries easily
- demo AJAX call with JSON response
- tries to follow PSR 1/2 coding guidelines, so we have quite clean code
- uses PDO for any database requests (so forget all the SQL injection / espacing stuff)
- killer feature: comes with a PDO debug function that emulates and shows your SQL statements
- commented code
- uses only native PHP code, so people don’t have to learn a framework
The requirements of MINI
- PHP 5.3+
- MySQL
- mod_rewrite activated
There’re copy & paste tutorials on how to activate mod_rewrite for Ubuntu 12.04 LTS, Ubuntu 14.04 LTS, EasyPHP on Windows, AMPPS on Windows/Mac OS, XAMPP for Windows and MAMP on Mac OS.
No mod_rewrite ? Then try TINY, the mod_rewrite-less version of MINI
Mod_rewrite can be installed installed easily on every server in the world. There’s absolutly no reason to rent “shared hosting” or “pre-setup servers” or similar stuff these days. Shared hosting is for beginners to upload HTML file etc. but definitly not for PHP development. DON’T DO SHARED HOSTING, it will only bring you into problems. You can get extremely cheap servers everything, without a contract, delivered in under 60 seconds. So “I cannot install X” or “I cannot setup mod_rewrite” is no valid excuse. Seriously. However, if you still don’t have mod_rewrite activated, then TINY, the mod_rewrite-less version of MINI might be worth a look. But keep in mind that this version is just a quick development tool, not intended for public release, see the Readme on GitHub for more information.
MINI 2 (the better one)
MINI has become quite popular, but I was never satisfied with the architecture, so I’ve written a similar version of MINI on top of the wonderful Slim micro framework and called it MINI2. Have a look on the official GitHub repo here.
The structure of MINI
We have the two main folders application (all application logic) and public (all the files that are accessable for the user). Note the .htaccess in the root of the script. Forget about any other folders that might exist (install stuff etc.) and the composer.json (totally optional, more on that later). For this paragraph it makes sense to open the files locally or on GitHub to read along.
What happens here ?
When the user enters the server, the .htaccess in the applications’s root folder will route him to /public/index.php. This also prevents any access to the application folder, making your application much more secure. The index.php loads the applications configs from /application/config/config.php (by default just the database logins and some automatically recognized path/URL settings) and MINI’s core logic from /application/core. It’s not important to know what exactly happens there for now.
Okay, MINI works like most other frameworks and uses URLs like this: example.com/songs, example.com/songs/editsong/17, example.com/subpage etc. instead of ugly messy index.php?xxx=111&yyy=222 URIs. The logic behind is simple: The first part after the domain (example.com) of the URL selects the controller(-file) in /application/controllers, the second part selects the method inside that file (so /songs/editsong will load /application/controllers/songs.php and call the editSong() method there). Extremely simple. If you only provide the first part, then the index() method will be called automatically, if you don’t provide the first part, then the home controller will be loaded and the index() method will be called automatically. In theory you can do whatever you want inside these methods to build your tool.
Let’s look at a real example: In the default demo setup the homepage (example.com) will load /applications/controllers/home.php (as explained above, code below in [1]) and call index() inside. In index() we simply load a header (/application/views/_templates/header.php), a footer (/application/views/_templates/footer.php) and some real content, in this case the content of /application/views/home/index.php (which is just a little bit of demo text). The principle behind should be clean, right ? Look into the files to get an idea.
Another example, this time one that uses data from the database: Look into /application/controllers/songs.php (code below in [2]): Same like before, but note this line:
$songs = $this->model->getAllSongs();
This simply fills a variable $songs with the results of the method getAllSongs() from the file /application/model/model.php! Self-explaning. In theory you can put whatever you want inside the model-file, usually methods that get, set or manipulate data in any way. In this case the method returns an array of data from the database. Now we can echo out the variable ($songs) inside the view file easily, like in listing [3] that shows how /application/views/songs/index.php looks like.
Extremely simple, right ? There are some more features, but the basic flow of MINI should be clear now. Feel free to experiment with the codebase and make sure you read the comments. Have a look into the header.php to see how CSS and JS is loaded and how links are generated. See the edit/delete/add-methods in the songs-controller to get an idea how CRUD operations are done here and how form handling flows.
Code listing [1]: /application/controllers/home.php
class Home extends Controller { public function index() { // load view files require APP . 'views/_templates/header.php'; require APP . 'views/home/index.php'; require APP . 'views/_templates/footer.php'; } // ... }
Code listing [2]: /application/controllers/songs.php
class Songs extends Controller { public function index() { // getting all songs and amount of songs $songs = $this->model->getAllSongs(); $amount_of_songs = $this->model->getAmountOfSongs(); // load view files require APP . 'views/_templates/header.php'; require APP . 'views/songs/index.php'; require APP . 'views/_templates/footer.php'; } // ... }
Code listing [3]: /application/views/songs/index.php (shortened)
<table> <?php foreach ($songs as $song) { ?> <tr> <td><?php if (isset($song->id)) echo htmlspecialchars($song->id, ENT_QUOTES, 'UTF-8'); ?></td> <td><?php if (isset($song->artist)) echo htmlspecialchars($song->artist, ENT_QUOTES, 'UTF-8'); ?></td> <td><?php if (isset($song->track)) echo htmlspecialchars($song->track, ENT_QUOTES, 'UTF-8'); ?></td> <td><a href="<?php echo URL . 'songs/deletesong/' . htmlspecialchars($song->id, ENT_QUOTES, 'UTF-8'); ?>">delete</a></td> <td><a href="<?php echo URL . 'songs/editsong/' . htmlspecialchars($song->id, ENT_QUOTES, 'UTF-8'); ?>">edit</a></td> </tr> <?php } ?> </table>
Installation of MINI
The installation of MINI is explained on GitHub for sure. Beside the classic manual installation there’s also a one-line installation script for Vagrant that will automatically install Apache, PHP, MySQL, PHPMyAdmin, git and Composer, set a chosen password in MySQL and PHPMyadmin and even inside the application code, download the Composer-dependencies (optional), activate mod_rewrite and edits the Apache settings, download the code from GitHub and run the demo SQL statements (for demo data). This is 100% automatic, you’ll end up after +/- 5 minutes with a fully running installation of MINI inside an Ubuntu 14.04 LTS Vagrant box.
Everything is explained on GitHub’s MINI readme page.